Surveys (Polls)
A survey allows you to ask a question to a channel. Channel participants can choose from the answers you specify, and the results can be viewed by everyone in the channel, or optionally only yourself. You can allow voters to select only one answer, or allow them to select multiple answers.
Here is a sample of what a complete poll implementation can look like:
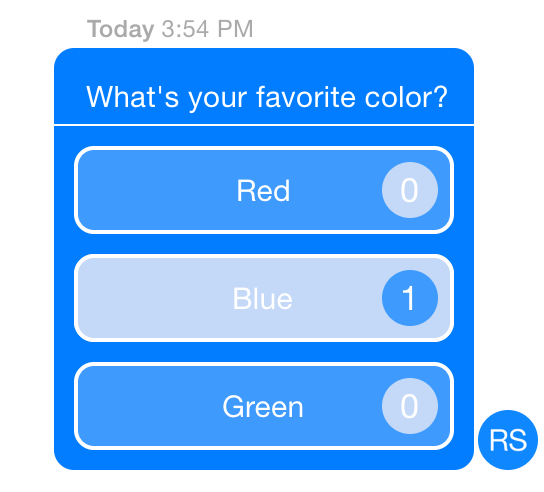
Create Poll
The following example creates a poll, MMXPoll, for particpants' favorite color.
//var myChannel = ...
// create a poll
var myPoll = MMXPoll(name: "Favorite Color", question: "What is your favorite color?", options: ["Red", "Blue", "Green"], hideResultsFromOthers: false, allowMultiChoice: true)
myPoll.publish(channel: myChannel, success: { (message) in
//Poll Created!
}, failure: { error in
//print("error \(error.localizedDescription)")
})
Listen for Incoming Poll Identifiers
Participants of the channel can listen for incoming poll identifiers, which contain a pollId we can use to get the poll object from the server.
func didReceiveMessage(notification: NSNotification) {
let tmp : [NSObject : AnyObject] = notification.userInfo!
let mmxMessage = tmp[MMXMessageKey] as! MMXMessage
// the incoming message contains a poll identifier
if let pollIdentifier = mmxMessage.payload as? MMXPollIdentifier where mmxMessage.contentType == MMXPollIdentifier.contentType {
let pollID = pollIdentifier.pollID
}
}
Get the poll object from the server using a pollId (poll identifier).
let pollID = ...;
MMXPoll.pollWithID(pollID, success: { poll in
// do something with poll object
}, failure: { error in
//print("error \(error.localizedDescription)")
})
A poll object contains all the information needed to display a poll in your user interface.
let name = myPoll.name // A user-friendly name of the poll.
let question = myPoll.question // The question this poll should answer.
let options = myPoll.options // A list of the available poll selection options.
let allowMultiChoice = myPoll.allowMultiChoice // If enabled, users can select more than one option.
let hideResultsFromOthers = myPoll.hideResultsFromOthers // If enabled, participants cannot obtain results from the poll, and will not receive {MMXPollAnswer} when a participant chooses a poll option. The poll creator can still obtain results using {PollWithID or PollFromMessage} or poll.refreshResults.
let extras = myPoll.extras // A user-defined object used to store arbitrary data will can accessed from a {Max.Poll} instance.
let endDate = myPoll.endDate // The date this poll ends. After a poll ends, users can no longer select options.
Poll Options
Participants can choose from one or more options.
// create an array of the PollOptions chosen by the current user.
let option1 = myPoll.options[0]
let option2 = myPoll.options[2]
let selectedOptions = [option1, option2]
myPoll.choose(selectedOptions, success: { (message) in
//Options chosen. Message will be sent if hideResultsFromOthers == false
}, failure: { (error) in
//print("error \(error.localizedDescription)")
})
Listen for Incoming Poll Answers
Channel participants listening for the incoming PollAnswer can view them in the user interface.
func didReceiveMessage(notification: NSNotification) {
let tmp : [NSObject : AnyObject] = notification.userInfo!
let mmxMessage = tmp[MMXMessageKey] as! MMXMessage
// the incoming message contains a poll answer
if let pollAnswer = mmxMessage.payload as? MMXPollAnswer where mmxMessage.contentType == MMXPollAnswer.contentType {
let pollID = pollAnswer.pollID
let pollName = pollAnswer.name // poll name
let pollQuestion = pollAnswer.question // poll question
let pollSelections = pollAnswer.currentSelection // the currentSelection property is a list of PollOption chosen by the sender
let senderUserId = mmxMessage.sender?.userID
}
}
If the current user has voted in the poll, the selected PollOption(s) can be obtained from the poll object.
let myPoll = ...
let myChoices = myPoll.myVotes // a list of PollOption selected by the current user
Obtain Poll Results
There are several ways to obtain poll results.
Get latest poll object from the server using a pollId:
var pollID = ...
MMXPoll.pollWithID(pollID, success: { poll in
// get the number of votes casts towards each option
let option1VoteCount = myPoll.options[0].count // returns the number of votes for option 1
let option1VoteText = myPoll.options[0].text // returns the value of option 1
let option2VoteCount = myPoll.options[1].count // returns the number of votes for option 2
let option2VoteText = myPoll.options[1].text // returns the value of option 2
}, failure: { error in
//print("error \(error.localizedDescription)")
})
Refresh existing poll object results:
var myPoll = ...;
myPoll.refreshResults(completion: { poll in
if let myPoll = poll {
let option1VoteCount = myPoll.options[0].count; // returns the number of votes for option 1
let option1VoteText = myPoll.options[0].text; // returns the value of option 1
} else {
//unsuccessful refresh
}
})
Use a listener to update existing poll objects results. This method saves a round-trip to the server, updating the poll results dynamically when a PollAnswer is received.
let pollAnswer = ...
myPoll.refreshResults(answer: pollAnswer)
If hideResultsFromOthers
was selected during poll creation, no poll answers will be sent out. Only the poll creator can obtain the results of the poll. In this case, the poll creator should check for results periodically using poll.refreshResults()
.
var myPoll = ...
myPoll.refreshResults(completion: { poll in
if let myPoll = poll {
let option1VoteCount = myPoll.options[0].count; // returns the number of votes for option 1
} else {
//unsuccessful refresh
}
})